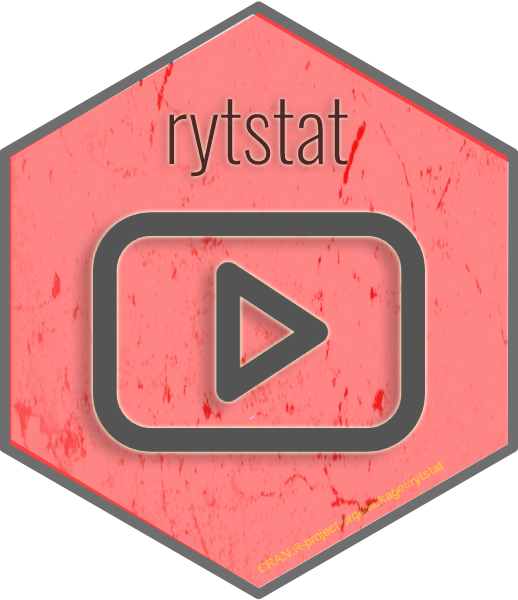
Returns a list of report types that the channel or content owner can retrieve. Each item in the list contains an id property, which identifies the report's ID, and you need this value to schedule a reporting job.
Source:R/ryt_get_report.R
reporting_api.Rd
By creating a reporting job, you are instructing YouTube to generate that report on a daily basis. The report is available within 24 hours of the time that the job is created.
Each resource in the response contains an id property, which specifies the ID that YouTube uses to uniquely identify the job. You need that ID to retrieve the list of reports that have been generated for the job or to delete the job.
Usage
ryt_get_report_types()
ryt_create_job(
report_type = c("channel_annotations_a1", "channel_basic_a2", "channel_cards_a1",
"channel_combined_a2", "channel_demographics_a1", "channel_device_os_a2",
"channel_end_screens_a1", "channel_playback_location_a2", "channel_province_a2",
"channel_sharing_service_a1", "channel_playback_location_a2", "channel_province_a2",
"channel_sharing_service_a1", "channel_subtitles_a2", "channel_traffic_source_a2",
"playlist_basic_a1", "playlist_device_os_a1", "playlist_playback_location_a1",
"playlist_province_a1", "playlist_traffic_source_a1")
)
ryt_get_job_list()
ryt_get_report_list(
job_id,
created_after = NULL,
start_time_at_or_after = NULL,
start_time_before = NULL
)
ryt_get_report(download_url)
ryt_get_report_metadata(job_id, report_id)
ryt_delete_job(job_id)
Arguments
- report_type
The type of report that the job creates. The property value corresponds to the id of a reportType as retrieved from the
ryt_get_report_types
function.- job_id
The ID that YouTube uses to uniquely identify the job that is being deleted. Use
ryt_get_job_list
.- created_after
If specified, this parameter indicates that the API response should only contain reports created after the specified date and time, including new reports with backfilled data. Note that the value pertains to the time that the report is created and not the dates associated with the returned data. The value is a timestamp in RFC3339 UTC "Zulu" format, accurate to microseconds. Example:
"2015-10-02T15:01:23.045678Z"
.- start_time_at_or_after
This parameter indicates that the API response should only contain reports if the earliest data in the report is on or after the specified date. Whereas the createdAfter parameter value pertains to the time the report was created, this date pertains to the data in the report. The value is a timestamp in RFC3339 UTC "Zulu" format, accurate to microseconds. Example:
"2015-10-02T15:01:23.045678Z"
.- start_time_before
This parameter indicates that the API response should only contain reports if the earliest data in the report is before the specified date. Whereas the createdAfter parameter value pertains to the time the report was created, this date pertains to the data in the report. The value is a timestamp in RFC3339 UTC "Zulu" format, accurate to microseconds. Example:
"2015-10-02T15:01:23.045678Z"
.- download_url
download URL, you can get it by
ryt_get_report_list
orryt_get_report_metadata
- report_id
The ID that YouTube uses to uniquely identify the report that is being retrieved. Use
ryt_get_report_list
Value
ryt_get_report_types: tibble with report types ryt_reports_create_job: No return value, called for side effects ryt_get_job_list: tibble with jobs metadata ryt_get_report_list: tibble with reports metadata ryt_get_report: tibble with report data ryt_get_report_metadata: list with report metadata ryt_reports_delete_job: No return value, called for side effects
See also
Examples
if (FALSE) {
# auth
ryt_auth('me@gmail.com')
# get reporting data
## create job
ryt_reports_create_job('channel_basic_a2')
## get job list
jobs <- ryt_get_job_list()
## get job report list
reports <- ryt_get_report_list(
job_id = jobs$id[1],
created_after = '2021-10-20T15:01:23.045678Z'
)
## get report data
data <- ryt_get_report(
download_url = reports$downloadUrl[1]
)
## delete job
ryt_reports_delete_job(jobs$id[1])
}